- Engineering
- 8 min read
- August 2023
11 Best Ways Node.js Powers High-Performance Web Servers
In the digital era, the performance of your web server can make or break your business. High-performance web servers are crucial for delivering content quickly and efficiently to your users, which is key to providing an optimal user experience, improving SEO rankings, and, ultimately, increasing your customer base. One technology that has emerged as particularly effective in powering high-performance web servers is Node.js.
What is Node.js?
Node.js is an open-source, cross-platform JavaScript runtime environment that executes JavaScript code outside a web browser. It enables JavaScript to be used for back-end development, allowing JavaScript applications to run server-side.
This is a significant departure from other backend technologies, as JavaScript was traditionally only used for front-end development.
Code snippet
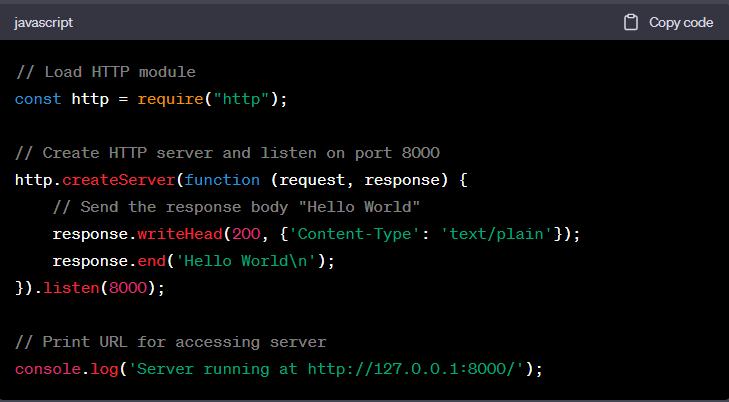
This code snippet creates a simple HTTP server using Node.js, which listens on port 8000 and responds with "Hello World."
What is web server performance?
Web server performance refers to the ability of a server to handle requests and deliver responses in the shortest time possible. "High performance" in the context of web servers means the server can handle many requests simultaneously without significant delays or downtimes.
According to a study by the Aberdeen Group, a one-second delay in page load time results in a 7% reduction in conversions, 11% fewer page views, and a 16% decrease in customer satisfaction.
Case study
Netflix, the world’s leading internet television network, transitioned from Java on the server side to Node.js, significantly improving performance. With the switch to Node.js, the start-up time for their application was reduced by 70%.
The architecture of Node.js
Node.js uses an event-driven, non-blocking I/O model, which makes it lightweight and efficient, perfect for data-intensive real-time applications that run across distributed devices. It is single-threaded, but because of its event-driven nature, it can handle more requests simultaneously than some other server-side technologies.
Event-driven architecture
An event-driven architecture means that the flow of the program is determined by events, such as user actions, sensor outputs, or messages from other programs or threads.
Code snippet
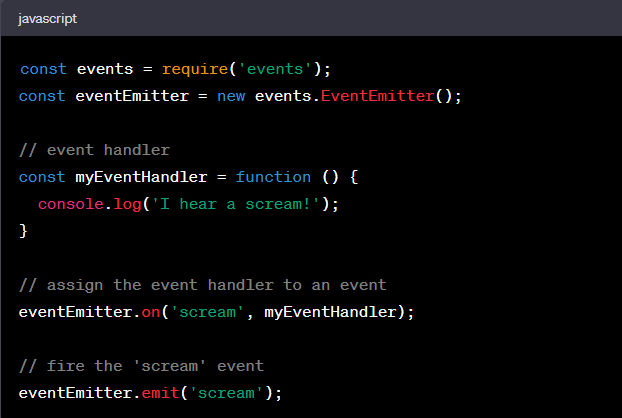
In this example, a simple event is created and emitted using Node.js’s event module.
Single-threaded nature and how it affects performance
Being single-threaded means that Node.js can only execute one command at a time. However, it doesn't mean it can only process one task simultaneously.
Node.js is designed to be non-blocking, meaning it can handle concurrent operations without multiple threads of execution, making it capable of handling many simultaneous connections.
PayPal reported a 35% decrease in the average response time for the same page when they moved from a Java-based application to a Node.js-based application.
This performance improvement was achieved, although the Node.js application was written in 33% fewer lines of code and was built by a team half the size.
How Node.js enhances web server performance
The architecture of Node.js is designed to optimize performance and scalability. Here are some of the key ways Node.js achieves high performance in web servers:
1. Single-threaded non-blocking IO model
Node.js uses a single-threaded, non-blocking IO model. This means that a single thread handles all requests and never waits for an IO operation to complete. Instead, it uses callbacks to signal the completion of an IO operation. This model optimizes performance and scalability, especially for IO-bound operations.
Code snippet
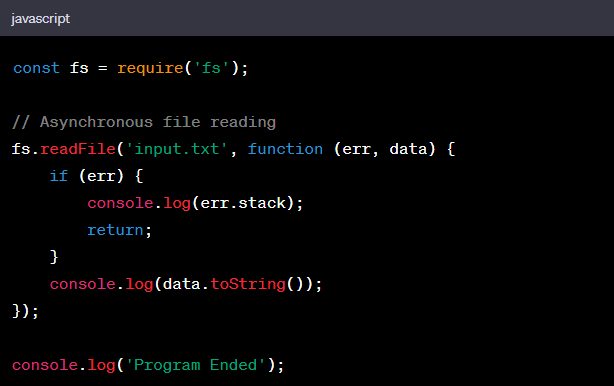
In this example, the program doesn’t wait for the file reading to complete and instead moves on to print “Program Ended,” demonstrating the non-blocking nature of Node.js.
2. V8 JavaScript engine
Node.js uses the V8 JavaScript engine developed by Google for the Chrome browser. V8 is extremely fast because it compiles JavaScript into native machine code instead of interpreting it or executing it as bytecode. This enhances server-side execution speed.
The performance of the V8 engine has been consistently improving over the years. For example, the Octane 2.0 benchmark shows a performance improvement of almost 100% from its first version in 2012 to its latest version.
3. Event loop
The event loop is a key component of the Node.js architecture. It allows Node.js to handle many connections simultaneously without multiple threads of execution by offloading operations and scheduling callbacks.
Code snippet
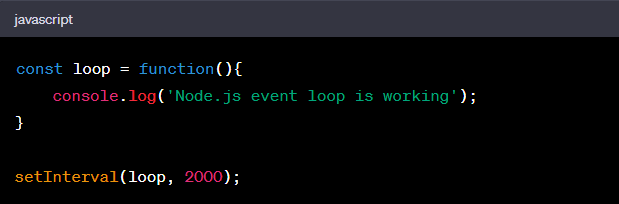
In this example, the setInterval function executes the loop function every 2000 milliseconds, demonstrating the event loop in action.
4. Streams
Streams in Node.js are used to handle data reading and writing more efficiently. They allow you to process data as soon as it’s available without waiting for the entire data chunk to be loaded.
Code snippet
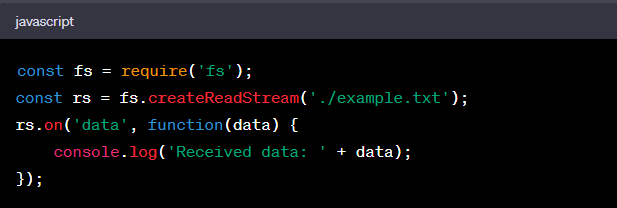
In this example, a readable stream is created, and data is processed as soon as it’s received, without waiting for the entire file to be read.
5. Cluster module
The cluster module in Node.js allows you to create multiple child processes that share the same server port. This enables you to utilize all the CPU cores of your machine, enhancing server performance.
Code snippet
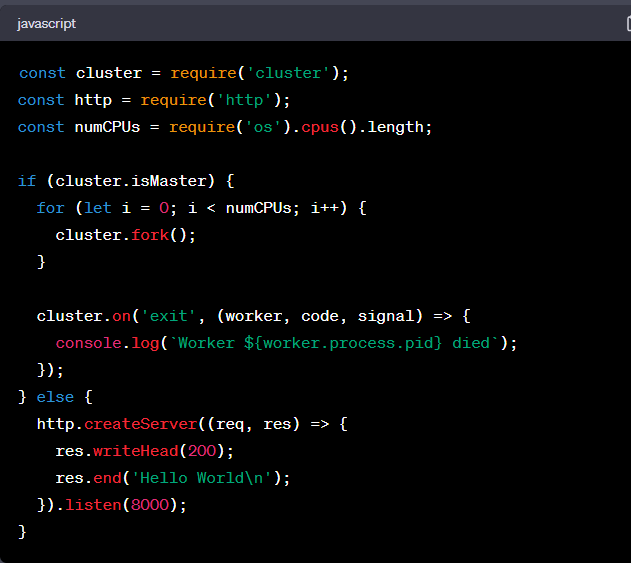
In this example, the master process forks multiple worker processes, each running its own HTTP server.
6. NPM (Node Package Manager)
NPM is the package manager for Node.js. It provides a vast and comprehensive package registry for developers to find and install modules to enhance their application’s functionality and performance.
As of 2021, over 1.3 million packages are available on the NPM registry, making it the largest software registry in the world.
7. Microservices architecture
Node.js facilitates the implementation of microservices architecture, an architectural style that structures an application as a collection of loosely coupled, independently deployable services. This enhances application structure and performance.
Uber uses a microservices architecture, with Node.js as a key technology. This has helped them scale their application to handle millions of daily rides.
8. Asynchronous nature
The asynchronous nature of Node.js means that IO operations such as network requests, database access, and file access do not block the execution of other operations. This helps in handling multiple operations simultaneously.
Code snippet
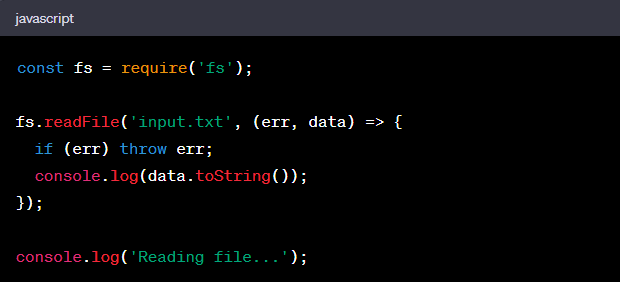
In this example, the fs.readFile function is non-blocking, and the program continues to execute, printing “Reading file…” before the file is completely read.
9. Lightweight
Node.js is lightweight because it doesn’t use multiple threads to handle requests and uses an event-driven architecture. This means that it doesn’t consume many system resources, improving performance.
10. WebSockets
Websockets provide a full-duplex communication channel over a single, long-lived connection. This allows for real-time two-way communication between the client and the server, which can significantly improve the performance of web applications.
Code snippet
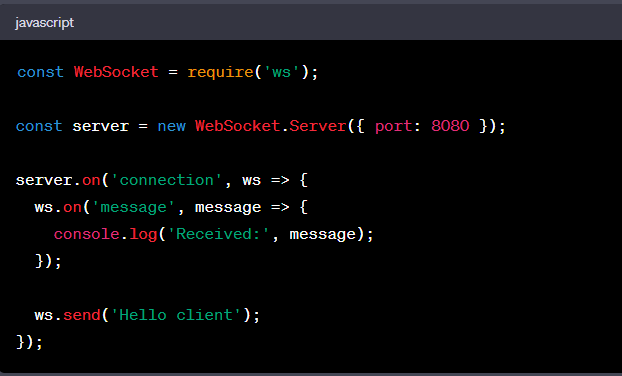
In this example, a simple WebSocket server is created using the ws library. The server listens for messages from the client and sends a message to the client upon connection.
11. Caching
Node.js supports caching of modules. Once a module is loaded, it is cached in the application memory, meaning the next time the module is required, it is not re-loaded from the file system, reducing redundant processing.
Code snippet
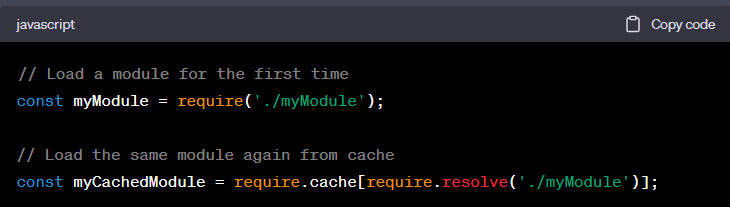
In this example, the myModule module is loaded for the first time and then retrieved from the cache using 'require.cache'.
Real-world case studies of high-performance web servers powered by Node.js
Here are some real-world examples of high-performance web servers powered by Node.js:
1. PayPal
PayPal is a global online payment system that supports online money transfers and serves as an electronic alternative to traditional paper methods like checks and money orders. They moved from Java to Node.js for their web applications, resulting in a 35% decrease in the response time of their application.
According to a PayPal Engineering blog post, the applications built with Node.js served double the number of requests per second compared to their previous Java application, with a 35% decrease in the average response time.
2. LinkedIn
LinkedIn, the world’s largest professional networking site, shifted its mobile app backend from Ruby on Rails to Node.js. This move led to a significant improvement in the app’s performance.
According to a LinkedIn Engineering blog post, the shift to Node.js resulted in the application being 20 times faster and using only a fraction of the resources.
3. Uber
Uber, the ride-hailing giant, uses Node.js in its massive microservices architecture. It has helped them handle their extremely high demand by efficiently scaling their application.
As mentioned earlier, Uber uses a microservices architecture with Node.js as a key technology. This architecture helps them scale their application to handle millions of daily rides.
The non-blocking IO model and the event loop of Node.js are crucial in handling many simultaneous connections with minimal overhead.
4. Netflix
Netflix, the world's leading internet entertainment service, uses Node.js for its web applications. This has helped them in reducing the startup time of their application.
According to a Netflix Tech Blog post, using Node.js reduced the application startup time by 70%.
5. eBay
eBay, a multinational e-commerce corporation, uses Node.js for its web applications. It has helped them maintain a high-performance application while handling millions of live connections.
According to an eBay Tech Blog post, eBay wanted to make their application more real-time and needed to handle a large number of live connections. Node.js was chosen because of its non-blocking IO model and event-driven architecture.
This enabled eBay to handle many connections with minimal server resources.
Supercharge Your Web Server with Node.js
Ready to supercharge your web server's performance with Node.js? Don't miss out on the opportunity to boost your user experience, SEO rankings, and customer base. Get started today by exploring the power of Node.js for your business. Contact Rapidops now for expert guidance and solutions to elevate your web server's performance!
Frequently asked questions about Node.js
Yes, Node.js can handle high traffic due to its non-blocking IO model, event loop, and cluster module support, which allows it to handle many simultaneous connections with minimal overhead.
Node.js is one of the fastest backend technologies due to its event-driven architecture, single-threaded non-blocking IO model, and V8 JavaScript engine, which enable fast server-side execution speed and high-performance asynchronous processing.
Yes, Node.js is suitable for large-scale applications as it supports microservices architecture, which allows structuring the application as a collection of loosely coupled, independently deployable services, enhancing application structure and performance.